Web3.js in 2 Minuten erklärt
- blockliv3
- 21. Juni 2022
- 2 Min. Lesezeit
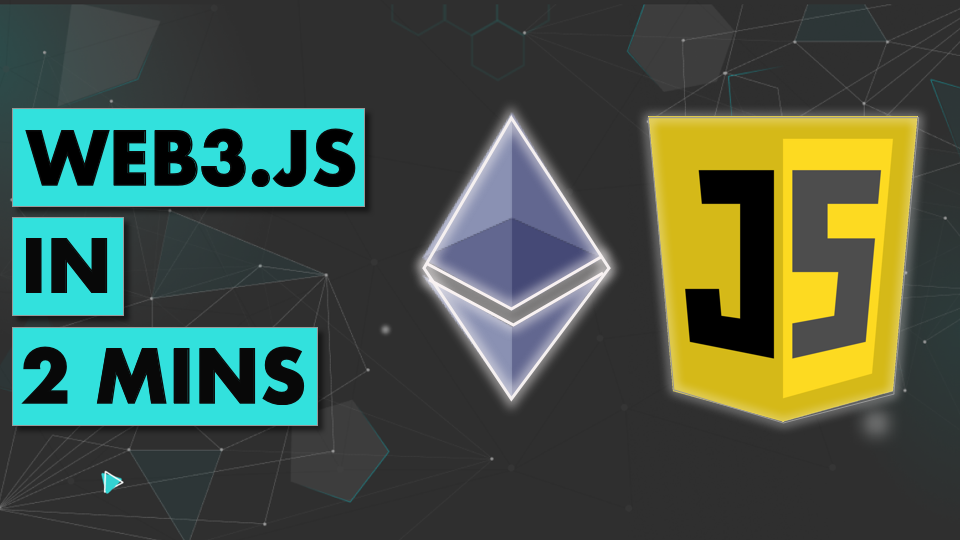
If you want to interact with a smart contract on Ethereum, the API of Ethereum is complicated. To interact with a smart contract, you have to use a couple of hexadecimal parameters, and it’s confusing. In this article, I will give you a quick intro to Web3.js, a library that makes it super easy to use the Ethereum API and interact with smart contracts. Beside Ethereum, Web3 can also be used with all Blockchains based on the Ethereum technology like Binance Smart Chain and Polygon.
Web3.js is a Javascript library written in NodeJS. You can use it server-side, on in a web browser. When you look for tutorials, make sure it’s for Web3 1.0 or above, because the syntax for the older versions is outdated. To use web3.js, you need to have a recent version of NodeJS.You start by installing web3 in your project:
npm install web3
In your script, you import web3.js:
const Web3 = require('web3');
Web3.js needs to connect to an Ethereum node. Running an Ethereum node is complex. By using a free account on Infura, you will get an url to connect to an Ethereum node. You can use this url to instantiate web3:
const web3 = new Web3('https://mainnet.infura.io/v3/ed18016b210c4a1baf828458bd16feb0');
With this web3 object, you can interact with the ethereum network.Next, you will need to add your private key to be able to send transactions:
web3.eth.accounts.wallet.add('0x55d0380decced82a2198582e541a129e8b86937c30a1c51bbb5327b9f00c6aa4');
To get the ether balance of an address, you can use the getBalance() method:
web3.eth.getBalance('0xFA17F09e8464Bd0c592Daf09bf285B5cD72Bec3D').then(balance => console.log(balance));
To interact with a smart contract, you have to first instantiate a contract object. You have to pass 2 arguments, the ABI, and the address of the contract.
const contract = new web3.eth.Contract(abi, '0x6E62c6e07019Bc3db5554a8d602390C6CC0B7846');
The ABI is a json document that describes the interface of the smart contract. Once you have this contract object, you can read value from the smart contract by specifying the function you call, and the call method:
//smart contract has a read() method
contract.methods.read().call().then(result => console.log(result));
To send a transaction, you can use the sendTransaction() function on web3.eth. This is used for simple Ether transfers:
web3.eth.sendTransaction({from: '0xFA17F09e8464Bd0c592Daf09bf285B5cD72Bec3D', value: 1000})
Or you use the sendTransaction() function on a contract object. This is used to execute smart contract functions:
contract.methods.write().sendTransaction({from: '0xFA17F09e8464Bd0c592Daf09bf285B5cD72Bec3D'});
There are many other cool stuffs you can do with web3, like listening to events, or formatting Ether values, and you can checkout the documentation of Web3 for more details.
Web3 is just one small part of everything you need to learn to become a Blockchain developer. You still have to learn how to create Solidity smart contracts and decentralized applications. And you also need to figure out how you can leverage your Blockchain skills to get a job in the industry. You could learn everything by yourself…Or you could take my course 6 figures blockchain developer and save a massive amount of time.
Komentarze